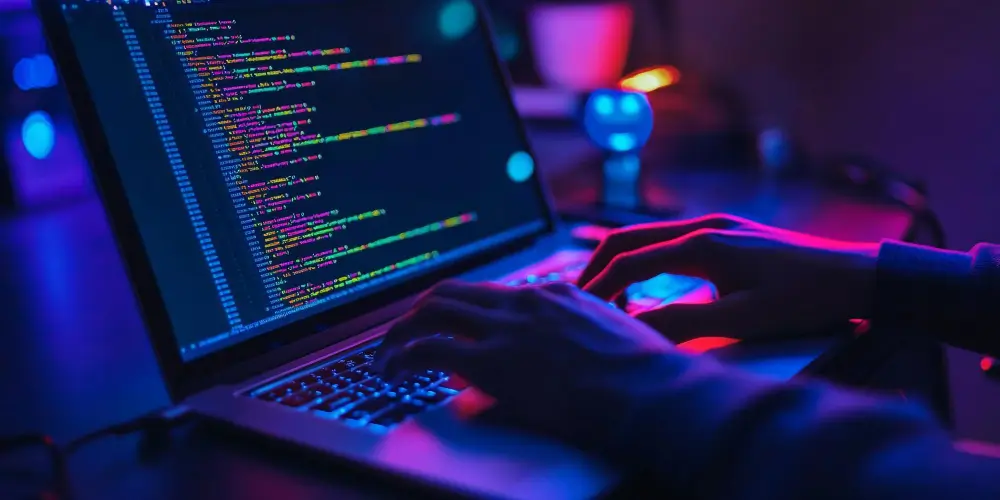
Yes, we start from the basics, but having some programming experience will be beneficial.
C++ Course
Our C++ programming course is designed to take you from a beginner to an advanced level in C++ development. C++ remains one of the most powerful and versatile programming languages, widely used in system/software development, game programming, embedded systems, and high-performance computing.
Key aspects of C++ and our course include:By completing this C++ course, you'll be well-equipped to develop a wide range of software applications and pursue careers in various tech sectors.
Ch. 1
Basic Classes
Ch. 2
Dynamic Allocation
Ch. 3
References
Ch. 4
Copy Constructor
Ch. 5
Streams
Ch. 6
Files Input & Output
Ch. 7
Strings
Ch. 8
Static Functions & Variables
Ch. 9
Friend Functions & Friend Classes
Ch. 10
This Pointer
Ch. 11
Operator Overloading
Ch. 12
Composition
Ch. 13
Inheritance
Ch. 14
Virtual Function Polymorphism
Ch. 15
Multiple Inheritance
Ch. 16
Pure Virtual Functions & ADT
Ch. 17
Runtime Type Identification
Ch. 18
Errors & Exceptions
Ch. 19
Design Patterns
Ch. 20
STL standard Template Library
Ch. 21
Vectors
Ch. 22
Iterators
Ch. 23
Templates
Ch. 24
Smart Pointers
Ch. 25
C++ for Real-Time Embedded Systems
Benny Cohen
Embedded Academy Founder and CEO
As a long-time veteran in the technology industry, Benny Cohen combines a deep passion for technology with extensive field experience. With a B.Sc. in Electronics Engineering and an M.Sc. in Communication Engineering, he has spent over 20 years developing software and hardware systems, including the last few years focusing on the cybersecurity industry. In addition to his role as the company founder & CEO, Benny also operates as a hands-on practitioner who specializes in penetration testing and has conducted significant security assessments for leading enterprises and security companies worldwide. His approachable teaching style and real-world expertise make learning both engaging and relevant.
Yes, we start from the basics, but having some programming experience will be beneficial.
We'll use popular IDEs like Visual Studio and CLion, but the concepts apply to any C++ development environment.
Yes, the course includes multiple coding projects, ranging from small exercises to a comprehensive final project.
Yes, we regularly update our content to reflect the latest C++ standards and best practices.
News, insights, and learning resources from Embedded Academy